What is List Comprehension in Python?
- Kelly Gloria
- Feb 13
- 3 min read
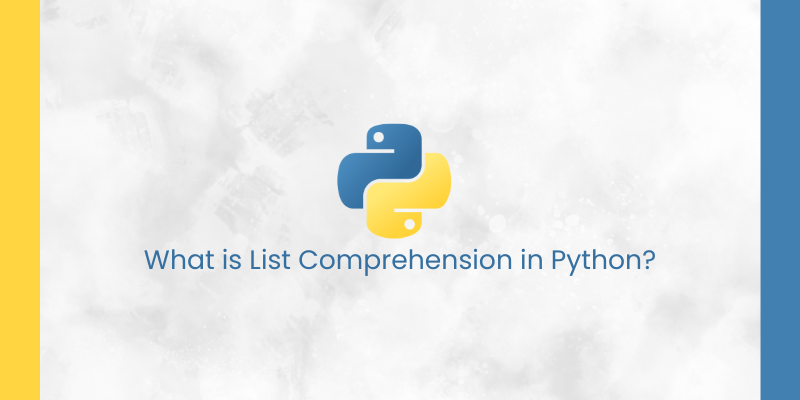
Python is an accessible programming language renowned for its accessibility. One of its excellent features, list comprehension, offers an efficient method for list creation without using traditional loops, making code more readable and efficient overall.
This article presents an in-depth examination of Python list comprehension, its workings and advantages; use cases and best practices are discussed as well.
What is list comprehension in Python?
List comprehension is an indispensable Python feature that enables more precise and refined data science list creation. It provides a shorter, more readable alternative to loops when creating lists.
The basic syntax of list comprehension is:
new_list = [expression for item in iterable if condition]
Components of List Comprehension
Expression: The operation or transformation applied to each element.
Iterable: The sequence (like a list, tuple, or range) from which elements are taken.
Condition (Optional): A filtering criterion that selects specific elements.
Example: Basic List Comprehension
Let's say we want to make a list of squares of numbers from 1 to 10.
Using a Traditional Loop:
squares = []
for num in range(1, 11):
squares.append(num ** 2)
print(squares)
Output:
[1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
Using List Comprehension:
squares = [num ** 2 for num in range(1, 11)]
print(squares)
Output:
[1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
As we can see, list comprehension reduces the lines of code while improving readability.
Advantages of List Comprehension
1. Concise and Readable
List comprehensions make code shorter and easier to understand compared to loops.
2. Performance Optimization
List comprehensions are faster than traditional loops since they are optimized internally in Python.
3. Reduced Code Complexity
By eliminating the need for explicit loops and temporary variables, list comprehensions lead to cleaner code.
Using Conditions in List Comprehension
Python list comprehensions allow us to filter elements based on conditions.
Example: Filtering Even Numbers
Using a Traditional Loop
even_numbers = []
for num in range(1, 11):
if num % 2 == 0:
even_numbers.append(num)
print(even_numbers)
Output:
[2, 4, 6, 8, 10]
Using List Comprehension
even_numbers = [num for num in range(1, 11) if num % 2 == 0]
print(even_numbers)
Output:
[2, 4, 6, 8, 10]
Here, the if condition filters out the odd numbers, keeping only even numbers.
Nested List Comprehension
Python also supports nested list comprehensions, allowing us to handle multi-dimensional lists efficiently.
Example: Flattening a List
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flat_list = [num for row in matrix for num in row]
print(flat_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
Using Functions Inside List Comprehension
We can use functions within list comprehensions to apply transformations to elements.
Example: Converting Strings to Uppercase
names = ["alice", "bob", "charlie"]
uppercase_names = [name.upper() for name in names]
print(uppercase_names)
Output:
['ALICE', 'BOB', 'CHARLIE']
Dictionary and Set Comprehensions
Apart from lists, Python also supports dictionary comprehension and set comprehension.
Dictionary Comprehension Example
numbers = [1, 2, 3, 4, 5]
square_dict = {num: num ** 2 for num in numbers}
print(square_dict)
Output:
{1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
Set Comprehension Example
numbers = [1, 2, 2, 3, 4, 4, 5]
unique_squares = {num ** 2 for num in numbers}
print(unique_squares)
Output:
{1, 4, 9, 16, 25}
Common Mistakes to Avoid
Overusing List Comprehension:
If a comprehension is too complex, it may reduce readability. Use traditional loops for better clarity.
Using Multiple Conditions Improperly:
Ensure that conditions are correctly structured to avoid syntax errors.
Memory Inefficiency with Large Data Sets:
When working with large data sets, consider using generator expressions (() instead of []) to save memory.
When to Use List Comprehension
✅ Use list comprehension when:
You need to create lists in a single line.
The transformation or filtering is simple.
Code readability is a priority.
❌ Avoid list comprehension when:
The logic is too complex.
Readability is compromised.
Conclusion
List comprehension in Python offers an effective and straightforward method for quickly creating lists in an intuitive syntax that improves readability, increases performance and simplifies code. By using python training course conditions, functions, and even nested structures, developers can manipulate data effortlessly.
Mastering list comprehension is key to creating clean and efficient Pythonic code. But using it correctly to maintain clarity and avoid unnecessary complexity is paramount.
Comments